Cloud Tinkerers
Where Cloud Pros and Enthusiasts Connect
A community-driven hub for cloud engineers, DevOps pros, and system admins. We dive deep into AWS, Azure, Docker, Kubernetes, and all things cloud. Whether you're troubleshooting, building, or just tinkering—you're in the right place.
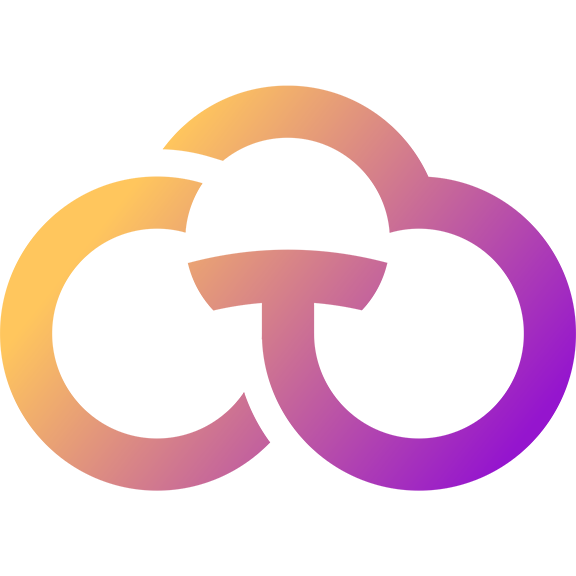
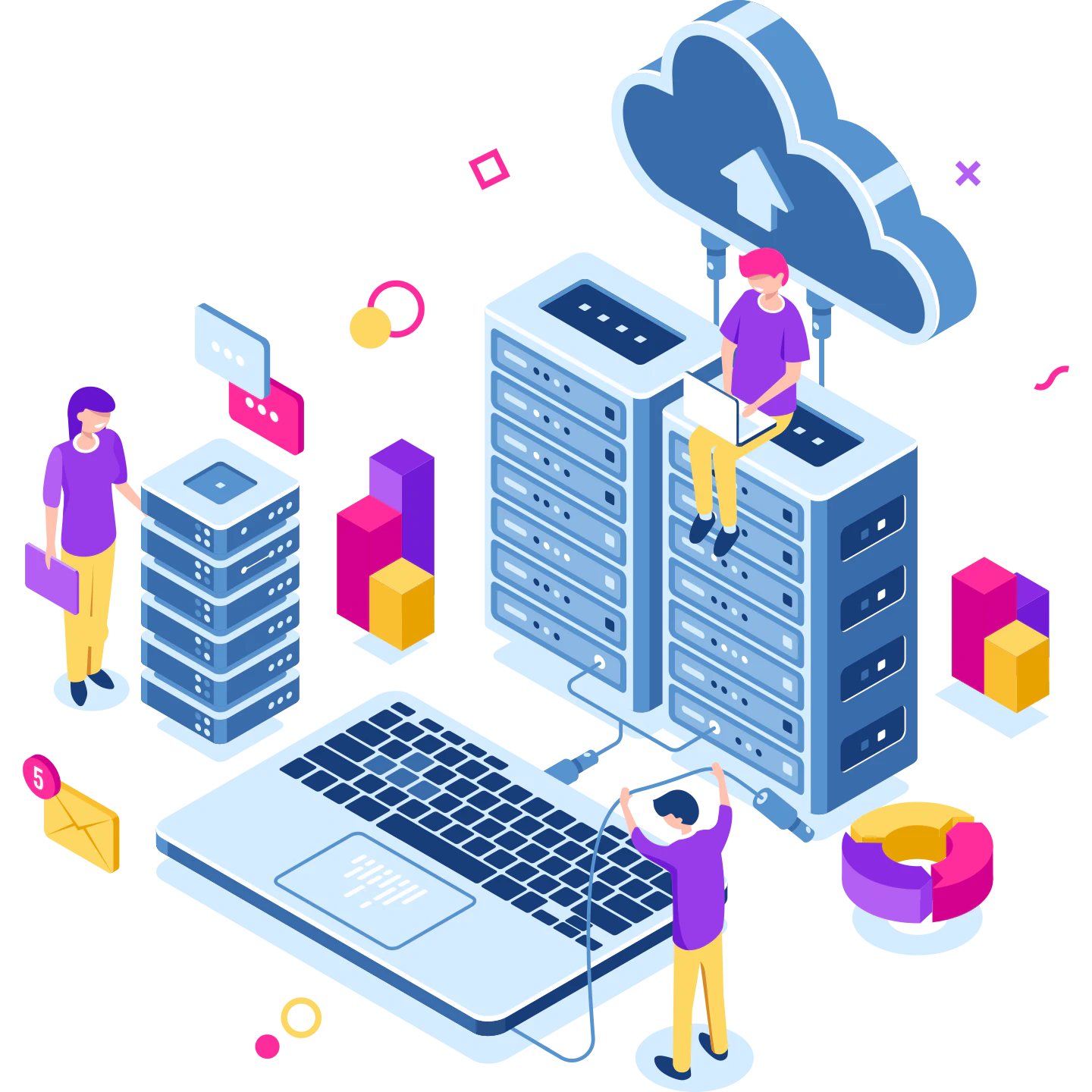
The Tinkerer's Mindset
Cloud computing isn’t just about servers—it’s an ever-evolving space that thrives on hands-on learning. Cloud Tinkerers is a community for those who love to experiment, break things, fix them, and share insights. Whether you're fine-tuning CI/CD pipelines, managing infrastructure, or exploring Kubernetes, you'll find like-minded professionals here.
Cloud Tinkerers is a space where cloud professionals learn, mentor, and solve real-world challenges. Linux, AWS, Terraform, and CI/CD, whatever it is, we help you grow.
Let’s build, learn, and solve together. 🚀
Check out our articles
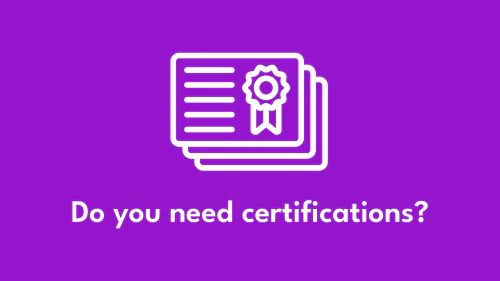
Will certifications slingshot your career forward?
Conor
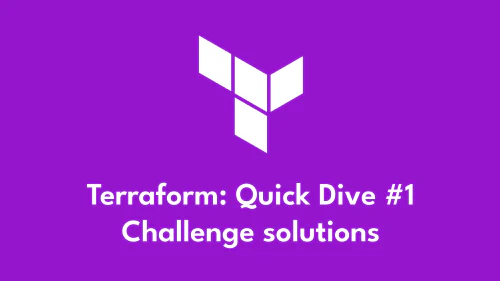
Discover how to get started with Terraform in AWS.
Conor
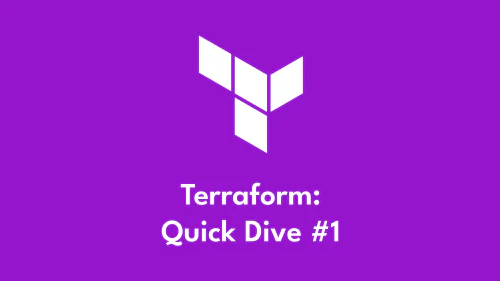
Discover how to get started with Terraform in AWS.
Conor